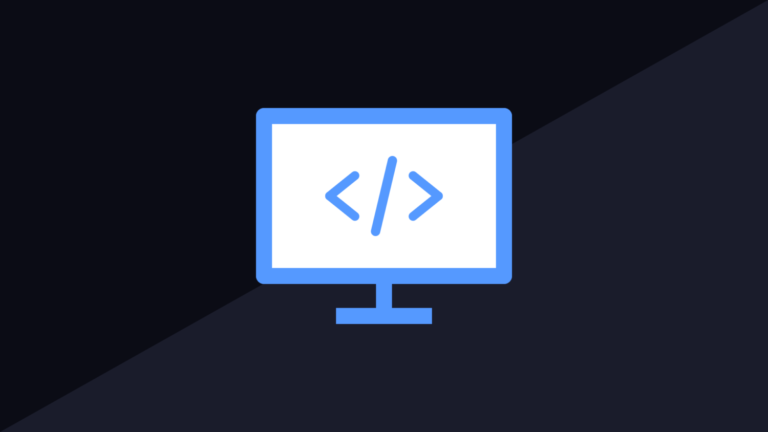
Performing analysis on content that a user is typing is a common requirement for interfaces. A simple word counter, for example, will need to bind to an event so that it can scan the text when the user has modified the data. There are ways to do this using jQuery, but what if you want to do it with TinyMCE? This guide will show you how to call an event when the user has finished typing with the TinyMCE editor.
The main reason for logic like this is to be able to run a function but wait until the user has given all of the information you need. There is no point in calling the function every time they lift up a key. The user will likely type faster than the code can execute. This is why it is important to wait until they have finished. Unfortunately, there is no event you can tap into that will trigger when the user has finished typing in TinyMCE. It is very easy to implement this on your own though.
The first part of this will require you to add code to handle the keyup event. This is done during the init of the framework. The “setup” property of the init object will allow you to handle various events. KeyUp is one of these. Tapping into this event is only one part of the process. You will need to also add additional logic to prevent the resulting function being called every time the user types a letter.
People have written lots of libraries and extension methods to handle delays in javascript. There is already some simple functionality built-in under the timeout objects as part of the editor framework. Declare a global timer before you load up TinyMCE. Each time the key up event is fired, reset the timer. This will allow you to define a specific amount of time to wait after the user has typed the last key.
Most people will press a key more than once per second while typing. Setting the timeout to one second seems like a save number to go with. The timer works with milliseconds. Entering 1000ms will count as 1 second. The code below can be used to call a function called “DoSomething” 1 second after the user has finished typing something into the TinyMCE editor.
var keypupTimer; tinymce.init({ selector:'#myeditor', setup : function(ed) { ed.on('KeyUp', function (e) { clearTimeout(keypupTimer); keypupTimer = setTimeout(DoSomething, 1000); }); } }); function DoSomething() { var editorText = tinyMCE.activeEditor.getContent(); }
You can rename the DoSomething function to whatever you like. Since you will more than likely be doing some kind of analysis on the content the user is typing, I have added code to get the editor text inside this method. If you do not need it, you can remove it. If the user has a very large document written, the call to get the text from the active editor could slow the page down.